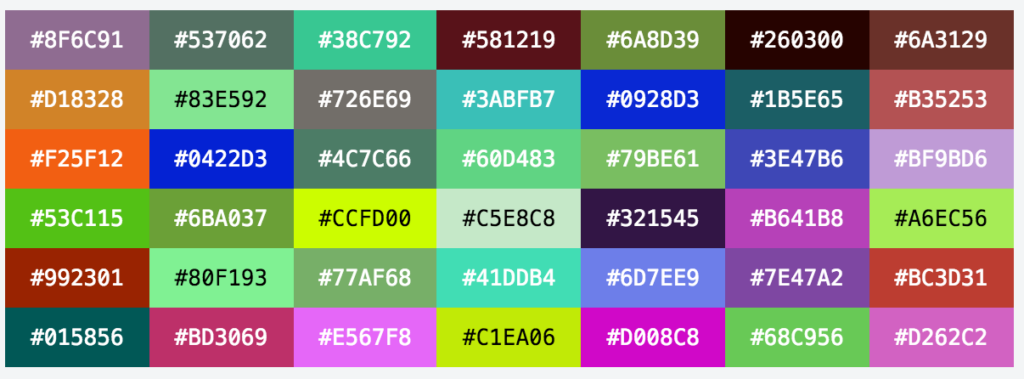
要如何取得某顏色的反差色?
使用太多顏色太花俏,使用二極化黑與白,效果會更好,上圖是進階版,指定 bw=true。
解法:How can I generate the opposite color according to current color?
https://stackoverflow.com/questions/35969656/how-can-i-generate-the-opposite-color-according-to-current-color
This is how I’d do it:
- Convert HEX to RGB
- Invert the R,G and B components
- Convert each component back to HEX
- Pad each component with zeros and output.
function invertColor(hex) {
if (hex.indexOf('#') === 0) {
hex = hex.slice(1);
}
// convert 3-digit hex to 6-digits.
if (hex.length === 3) {
hex = hex[0] + hex[0] + hex[1] + hex[1] + hex[2] + hex[2];
}
if (hex.length !== 6) {
throw new Error('Invalid HEX color.');
}
// invert color components
var r = (255 - parseInt(hex.slice(0, 2), 16)).toString(16),
g = (255 - parseInt(hex.slice(2, 4), 16)).toString(16),
b = (255 - parseInt(hex.slice(4, 6), 16)).toString(16);
// pad each with zeros and return
return '#' + padZero(r) + padZero(g) + padZero(b);
}
function padZero(str, len) {
len = len || 2;
var zeros = new Array(len).join('0');
return (zeros + str).slice(-len);
}
Advanced Version:
This has a bw
option that will decide whether to invert to black or white; so you’ll get more contrast which is generally better for the human eye.
function invertColor(hex, bw) {
if (hex.indexOf('#') === 0) {
hex = hex.slice(1);
}
// convert 3-digit hex to 6-digits.
if (hex.length === 3) {
hex = hex[0] + hex[0] + hex[1] + hex[1] + hex[2] + hex[2];
}
if (hex.length !== 6) {
throw new Error('Invalid HEX color.');
}
var r = parseInt(hex.slice(0, 2), 16),
g = parseInt(hex.slice(2, 4), 16),
b = parseInt(hex.slice(4, 6), 16);
if (bw) {
// https://stackoverflow.com/a/3943023/112731
return (r * 0.299 + g * 0.587 + b * 0.114) > 186
? '#000000'
: '#FFFFFF';
}
// invert color components
r = (255 - r).toString(16);
g = (255 - g).toString(16);
b = (255 - b).toString(16);
// pad each with zeros and return
return "#" + padZero(r) + padZero(g) + padZero(b);
}
實作的結果1:只加入陰影
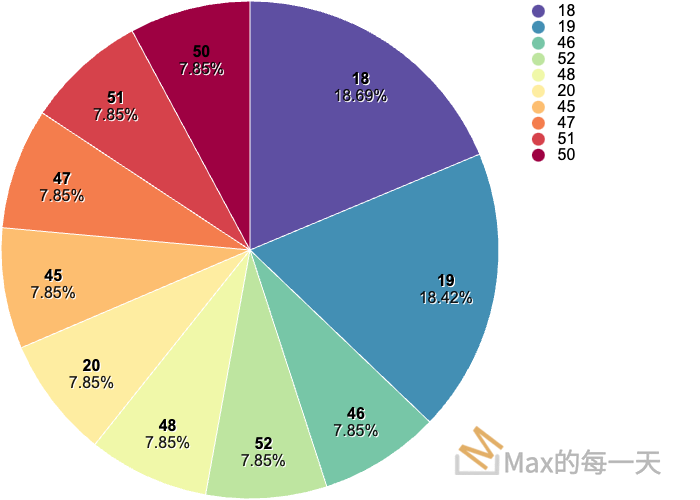
實作的結果2:依舊填入的顏色判斷最佳前景色, 並加入陰影
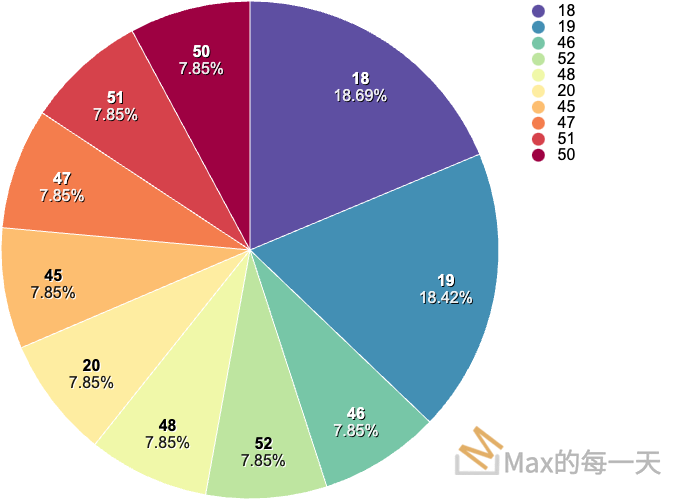
實作的結果3:依舊填入的顏色判斷最佳前景色, 效果就滿好的了。
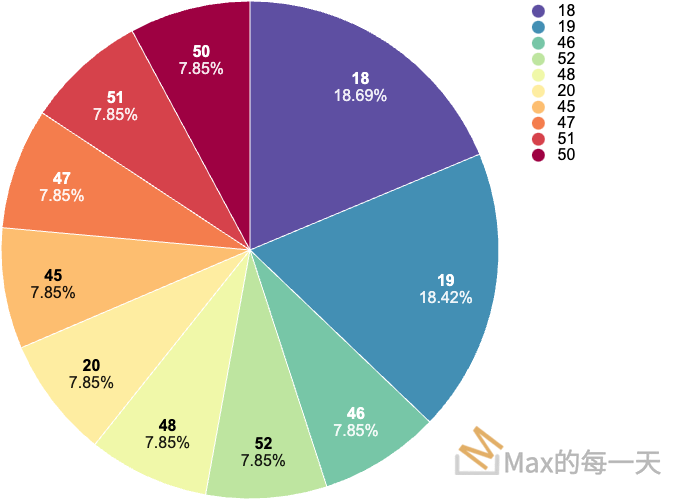