原本的需求是想知道圖片中一個4角形的內容值,剛好看到一個實用的範例。似乎 OpenCV 和 numpy 變成必學的項目了,好多專案都在使用。
How to get pixel values inside of a rectangle within an image
https://stackoverflow.com/questions/58790535/how-to-get-pixel-values-inside-of-a-rectangle-within-an-image
You can do that with Python/OpenCV by first drawing a white filled polygon on black background as a mask from your four points. The use np.where to locate and then print all the points in the image corresponding to the white pixels in the mask.
Input:
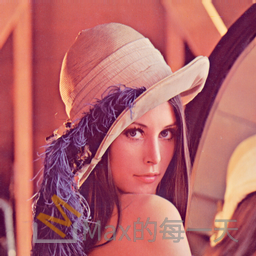
import cv2
import numpy as np
# read image
image = cv2.imread('lena.png')
# create mask with zeros
mask = np.zeros((image.shape), dtype=np.uint8)
# define points (as small diamond shape)
pts = np.array( [[[25,20],[30,25],[25,30],[20,25]]], dtype=np.int32 )
cv2.fillPoly(mask, pts, (255,255,255) )
# get color values
values = image[np.where((mask == (255,255,255)).all(axis=2))]
print(values)
# save mask
cv2.imwrite('diamond_mask.png', mask)
cv2.imshow('image', image)
cv2.imshow('mask', mask)
cv2.waitKey()
Mask:
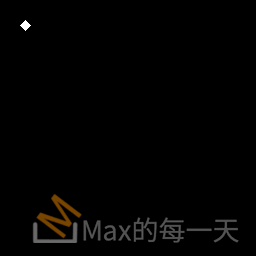
Results:
[[108 137 232]
[104 134 232]
[108 136 231]
[106 134 231]
[109 133 228]
[108 136 229]
[109 137 230]
[110 135 232]
[103 126 230]
[112 134 228]
[114 136 228]
[111 138 230]
[110 137 233]
[103 135 234]
[103 126 230]
[101 120 226]
[108 137 230]
[112 133 228]
[114 136 227]
[115 139 232]
[112 137 232]
[105 134 233]
[102 128 232]
[ 98 119 226]
[ 93 105 220]
[108 139 230]
[110 137 230]
[112 135 230]
[113 135 230]
[111 138 231]
[112 139 232]
[109 134 233]
[101 128 232]
[100 120 224]
[ 90 104 221]
[ 87 95 211]
[111 138 229]
[109 135 231]
[109 136 230]
[113 141 233]
[110 139 233]
[105 136 234]
[101 127 232]
[ 95 117 225]
[ 90 107 220]
[110 137 231]
[110 138 231]
[107 140 236]
[110 139 233]
[104 135 234]
[105 130 231]
[ 92 116 227]
[114 141 234]
[112 142 235]
[111 140 235]
[111 138 234]
[110 132 232]
[114 140 234]
[108 140 233]
[107 134 233]
[107 140 235]]
換成 PIL 寫法:
How to read an image file as ndarray
from PIL import Image import numpy as np im = np.array(Image.open('data/src/lena_square.png')) print(im.dtype) # uint8 print(im.ndim) # 3 print(im.shape) # (512, 512, 3)