想要實作一個瀏覽file system 的功能, 需要列出某一個路徑下的檔案或目錄清單, 畫面:
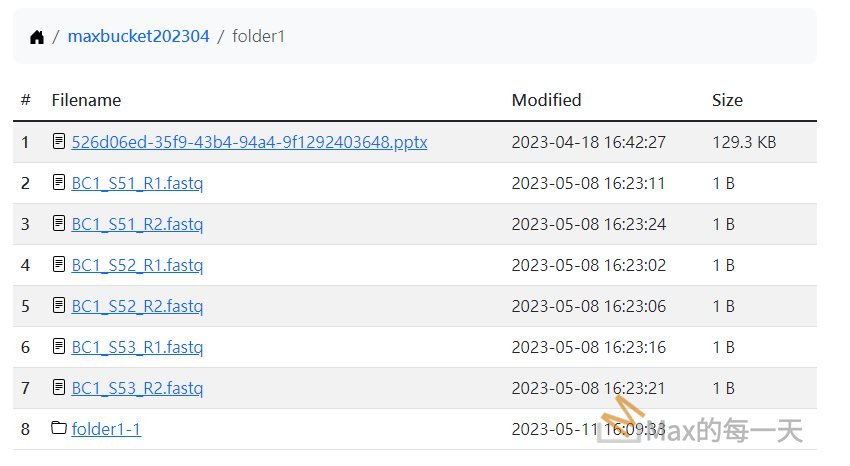
解法:
https://stackoverflow.com/questions/5694385/getting-the-filenames-of-all-files-in-a-folder
File folder = new File("your/path");
File[] listOfFiles = folder.listFiles();
for (int i = 0; i < listOfFiles.length; i++) {
if (listOfFiles[i].isFile()) {
System.out.println("File " + listOfFiles[i].getName());
} else if (listOfFiles[i].isDirectory()) {
System.out.println("Directory " + listOfFiles[i].getName());
}
}
解法2:
https://stackabuse.com/java-list-files-in-a-directory/
public class ListFilesRecursively {
public void listFiles(String startDir) {
File dir = new File(startDir);
File[] files = dir.listFiles();
if (files != null && files.length > 0) {
for (File file : files) {
// Check if the file is a directory
if (file.isDirectory()) {
// We will not print the directory name, just use it as a new
// starting point to list files from
listDirectory(file.getAbsolutePath());
} else {
// We can use .length() to get the file size
System.out.println(file.getName() + " (size in bytes: " + file.length()+")");
}
}
}
}
public static void main(String[] args) {
ListFilesRecursively test = new ListFilesRecursively();
String startDir = ("D:/Programming");
test.listFiles(startDir);
}
}
output:
yout-file-name.txt (size in bytes: 12345)