有一個按鈕是disabled, 希望有 checkbox 被勾的情況下, 自動啟用按鈕, 執行畫面:
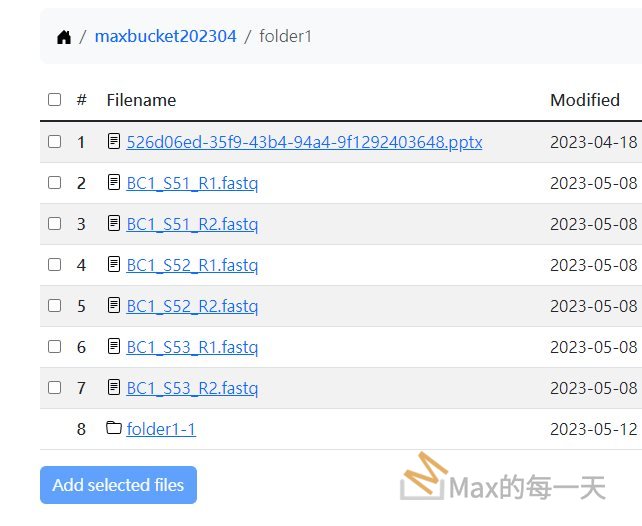
解法:
https://stackoverflow.com/questions/14800954/how-to-check-if-all-checkboxes-are-unchecked
var textinputs = document.querySelectorAll('input[type=checkbox]');
var empty = [].filter.call( textinputs, function( el ) {
return !el.checked
});
if (textinputs.length == empty.length) {
alert("None filled");
return false;
}
解法2: Checkbox Check Event Listener
https://stackoverflow.com/questions/14544104/checkbox-check-event-listener
Multiple checkboxes
Here’s an example of a list of checkboxes. To select multiple elements we use querySelectorAll
instead of querySelector
. Then use Array.filter
and Array.map
to extract checked values.
// Select all checkboxes with the name 'settings' using querySelectorAll.
var checkboxes = document.querySelectorAll("input[type=checkbox][name=settings]");
let enabledSettings = []
/*
For IE11 support, replace arrow functions with normal functions and
use a polyfill for Array.forEach:
https://vanillajstoolkit.com/polyfills/arrayforeach/
*/
// Use Array.forEach to add an event listener to each checkbox.
checkboxes.forEach(function(checkbox) {
checkbox.addEventListener('change', function() {
enabledSettings =
Array.from(checkboxes) // Convert checkboxes to an array to use filter and map.
.filter(i => i.checked) // Use Array.filter to remove unchecked checkboxes.
.map(i => i.value) // Use Array.map to extract only the checkbox values from the array of objects.
console.log(enabledSettings)
})
});
<label>
<input type="checkbox" name="settings" value="forcefield">
Enable forcefield
</label>
<label>
<input type="checkbox" name="settings" value="invisibilitycloak">
Enable invisibility cloak
</label>
<label>
<input type="checkbox" name="settings" value="warpspeed">
Enable warp speed
</label>
Multiple checkboxes with jQuery
let checkboxes = $("input[type=checkbox][name=settings]")
let enabledSettings = [];
// Attach a change event handler to the checkboxes.
checkboxes.change(function() {
enabledSettings = checkboxes
.filter(":checked") // Filter out unchecked boxes.
.map(function() { // Extract values using jQuery map.
return this.value;
})
.get() // Get array.
console.log(enabledSettings);
});
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<label>
<input type="checkbox" name="settings" value="forcefield">
Enable forcefield
</label>
<label>
<input type="checkbox" name="settings" value="invisibilitycloak">
Enable invisibility cloak
</label>
<label>
<input type="checkbox" name="settings" value="warpspeed">
Enable warp speed
</label>