我有一個類似 stack 的 array , 想只保留 array 裡的前N筆.
使用的情境是有一個檔案瀏覽時的 nav, 當不是最後一個項目時, 要允許使用者pop 到目的的階層:
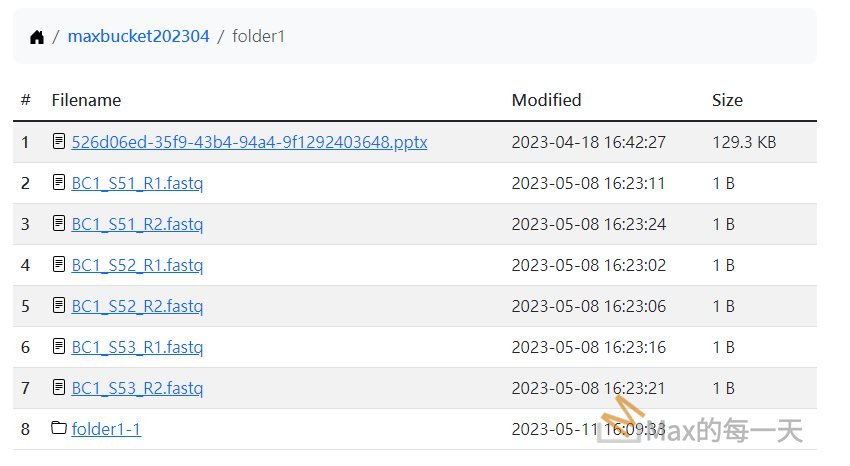
解法:
https://stackoverflow.com/questions/953071/how-to-easily-truncate-an-array-with-javascript
There is a slice method
let arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
arr = arr.slice(0, 4);
console.log(arr);
使用範例:
PS: 這個使用起來, 有一點點像 python 的 array 裡的用法, 例如: 負數指從右邊數過來.
const animals = ['ant', 'bison', 'camel', 'duck', 'elephant'];
console.log(animals.slice(2));
// Expected output: Array ["camel", "duck", "elephant"]
console.log(animals.slice(2, 4));
// Expected output: Array ["camel", "duck"]
console.log(animals.slice(1, 5));
// Expected output: Array ["bison", "camel", "duck", "elephant"]
console.log(animals.slice(-2));
// Expected output: Array ["duck", "elephant"]
console.log(animals.slice(2, -1));
// Expected output: Array ["camel", "duck"]
console.log(animals.slice());
// Expected output: Array ["ant", "bison", "camel", "duck", "elephant"]
跟 .slice() 很像的是多一個p 的 splice().
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/splice
使用範例:
Remove 0 (zero) elements before index 2, and insert "drum"
const myFish = ["angel", "clown", "mandarin", "sturgeon"];
const removed = myFish.splice(2, 0, "drum");
// myFish is ["angel", "clown", "drum", "mandarin", "sturgeon"]
// removed is [], no elements removed
Copy to Clipboard
Remove 0 (zero) elements before index 2, and insert "drum" and "guitar"
const myFish = ["angel", "clown", "mandarin", "sturgeon"];
const removed = myFish.splice(2, 0, "drum", "guitar");
// myFish is ["angel", "clown", "drum", "guitar", "mandarin", "sturgeon"]
// removed is [], no elements removed
Copy to Clipboard
Remove 1 element at index 3
const myFish = ["angel", "clown", "drum", "mandarin", "sturgeon"];
const removed = myFish.splice(3, 1);
// myFish is ["angel", "clown", "drum", "sturgeon"]
// removed is ["mandarin"]
Copy to Clipboard
Remove 1 element at index 2, and insert "trumpet"
const myFish = ["angel", "clown", "drum", "sturgeon"];
const removed = myFish.splice(2, 1, "trumpet");
// myFish is ["angel", "clown", "trumpet", "sturgeon"]
// removed is ["drum"]
Copy to Clipboard
Remove 2 elements from index 0, and insert "parrot", "anemone" and "blue"
const myFish = ["angel", "clown", "trumpet", "sturgeon"];
const removed = myFish.splice(0, 2, "parrot", "anemone", "blue");
// myFish is ["parrot", "anemone", "blue", "trumpet", "sturgeon"]
// removed is ["angel", "clown"]
Copy to Clipboard
Remove 2 elements, starting from index 2
const myFish = ["parrot", "anemone", "blue", "trumpet", "sturgeon"];
const removed = myFish.splice(2, 2);
// myFish is ["parrot", "anemone", "sturgeon"]
// removed is ["blue", "trumpet"]
Copy to Clipboard
Remove 1 element from index -2
const myFish = ["angel", "clown", "mandarin", "sturgeon"];
const removed = myFish.splice(-2, 1);
// myFish is ["angel", "clown", "sturgeon"]
// removed is ["mandarin"]
Copy to Clipboard
Remove all elements, starting from index 2
const myFish = ["angel", "clown", "mandarin", "sturgeon"];
const removed = myFish.splice(2);
// myFish is ["angel", "clown"]
// removed is ["mandarin", "sturgeon"]